Imagine this, you're coding away, building something truly amazing, but then BAM! You're hit with a wall of errors, inconsistencies, and messy code. That's where Lint Walker comes in. It's like your personal coding coach, helping you clean up your code and make it shine. Whether you're a beginner or a seasoned pro, lint walkers are essential tools that every developer should have in their toolkit.
Now, you might be wondering, "What exactly is a lint walker?" Well, buckle up, because we're about to dive deep into the world of lint walkers and why they matter so much in modern software development. Think of lint walkers as the grammar police for your code—making sure everything is neat, tidy, and error-free.
But here's the thing, lint walkers aren't just about fixing mistakes. They're about improving efficiency, enhancing readability, and ensuring your codebase is future-proof. In this article, we'll break down everything you need to know about lint walkers, from their basic functions to advanced use cases. So, grab a cup of coffee (or your favorite energy drink), and let's get started!
Table of Contents:
- What is Lint Walker?
- Why Lint Walker Matters
- How Lint Walker Works
- Top Lint Walker Tools
- Benefits of Using Lint Walker
- Lint Walker for JavaScript
- Lint Walker for Python
- Integrating Lint Walker into Your Workflow
- Common Mistakes to Avoid
- The Future of Lint Walker
What is Lint Walker?
Alright, let's start with the basics. A lint walker, also known as a linter, is a tool that analyzes your source code for potential errors, inconsistencies, and code quality issues. It's like having a second pair of eyes scanning your code to catch things you might have missed.
Lint walkers are particularly useful in large codebases where maintaining consistency can be a challenge. They help enforce coding standards, making your code more readable and maintainable. And trust me, when you're working on a project with multiple developers, having a lint walker is a game-changer.
Here's the kicker, lint walkers aren't just for one programming language. You can find lint walkers for almost every language out there, including JavaScript, Python, C++, and more. Each lint walker is tailored to the specific needs of the language it supports, ensuring that your code is optimized for that environment.
Why Lint Walker Matters
Let's face it, coding without a lint walker is like driving without a GPS. Sure, you might get where you're going eventually, but it's gonna take a lot longer and be way more stressful. Lint walkers matter because they help you catch issues early, before they become major problems.
For example, let's say you're working on a JavaScript project and you accidentally leave out a semicolon. Without a lint walker, that tiny mistake could cause your entire application to crash. But with a lint walker, it'll catch that error right away, saving you hours of debugging time.
Plus, lint walkers help you adhere to coding standards, which is crucial in collaborative environments. When everyone on your team follows the same coding conventions, it makes the code easier to read and understand. And let's be honest, nobody likes trying to decipher someone else's messy code.
How Lint Walker Works
Now that we know what lint walkers are and why they're important, let's talk about how they actually work. Lint walkers operate by scanning your code and comparing it against a set of predefined rules or standards. These rules can vary depending on the lint walker and the language it supports.
For instance, in JavaScript, a popular lint walker called ESLint checks your code against the ECMAScript standards. It looks for things like unused variables, missing semicolons, and inconsistent indentation. If it finds any issues, it'll flag them and provide suggestions on how to fix them.
Here's a quick rundown of the typical process:
- Scanning: The lint walker reads through your code line by line.
- Analysis: It compares your code against the set of rules it's configured with.
- Reporting: If it finds any issues, it generates a report detailing the problems and where they occur.
- Fixing: Some lint walkers can even automatically fix certain types of issues for you.
This whole process happens almost instantly, so you can get feedback on your code as you write it. It's like having a real-time code review, which is pretty darn cool if you ask me.
Top Lint Walker Tools
Now that you know how lint walkers work, you're probably wondering which ones you should use. Well, here's a list of some of the top lint walker tools out there:
- ESLint: The go-to lint walker for JavaScript developers. It's highly configurable and supports a wide range of plugins.
- flake8: A popular lint walker for Python that combines the power of PyFlakes, pycodestyle, and Ned Batchelder's McCabe script.
- Pylint: Another great option for Python developers, Pylint provides a more comprehensive analysis of your code.
- Stylelint: Perfect for CSS and SCSS developers, Stylelint helps you maintain consistent styles across your projects.
- GolangCI-Lint: If you're working with Go, this lint walker is a must-have. It combines multiple linters into one convenient tool.
Each of these tools has its own strengths and weaknesses, so it's important to choose the one that best fits your needs. And don't worry, we'll dive deeper into some of these tools later on in the article.
Benefits of Using Lint Walker
By now, you're probably convinced that lint walkers are pretty awesome. But just in case you need a little more convincing, here's a list of the top benefits of using a lint walker:
- Error Prevention: Catch errors early before they become major issues.
- Code Consistency: Ensure that your code follows consistent standards across your team.
- Improved Readability: Clean, well-formatted code is easier to read and understand.
- Time-Saving: Spend less time debugging and more time building amazing things.
- Enhanced Collaboration: Make it easier for other developers to work with your code.
And let's not forget, using a lint walker can actually make coding more fun. Who doesn't love the satisfaction of writing clean, error-free code? It's like leveling up in a video game every time you fix an issue.
Lint Walker for JavaScript
JavaScript is one of the most popular programming languages out there, and for good reason. But with great power comes great responsibility, and that's where lint walkers come in. Let's take a closer look at some of the best lint walkers for JavaScript.
ESLint is hands down the most popular lint walker for JavaScript. It's highly customizable, supports a wide range of rules, and integrates seamlessly with most code editors. You can even configure it to work with different JavaScript frameworks like React, Angular, and Vue.
Here's a quick example of how you might set up ESLint in your project:
First, install ESLint using npm:
npm install eslint --save-dev
Then, run the initialization script:
npx eslint --init
And just like that, you're ready to start linting your JavaScript code!
Lint Walker for Python
Python is another language that benefits greatly from lint walkers. With its emphasis on readability and simplicity, having a lint walker can really enhance your Python development experience.
Two of the most popular lint walkers for Python are flake8 and Pylint. Both tools offer similar functionality, but they have their own unique features that set them apart.
For example, flake8 is great for catching syntax errors and style violations, while Pylint provides more in-depth analysis of your code. You can even use them together for maximum effectiveness.
Here's how you might set up flake8 in your Python project:
First, install flake8 using pip:
pip install flake8
Then, run it on your code:
flake8 your_code.py
Simple, right? And just like that, you're on your way to writing cleaner, more efficient Python code.
Integrating Lint Walker into Your Workflow
Now that you know all about lint walkers, it's time to start integrating them into your development workflow. The good news is, most lint walkers are easy to set up and use. You can even configure them to run automatically every time you save a file or commit changes to your repository.
For example, if you're using Visual Studio Code, you can install extensions for ESLint, flake8, or Pylint that will automatically lint your code as you write it. This real-time feedback can be incredibly helpful in catching issues early.
Additionally, you can set up continuous integration (CI) pipelines to run lint checks on your code before it gets merged into the main branch. This ensures that only clean, error-free code makes it into your production environment.
Common Mistakes to Avoid
Even with all the benefits of lint walkers, there are still some common mistakes that developers make when using them. Here are a few to watch out for:
- Over-reliance: While lint walkers are great tools, they shouldn't replace your own code reviews. Always take the time to review your code manually as well.
- Ignoring Warnings: Just because a lint walker flags something doesn't mean you can ignore it. Take the time to understand why it's flagging the issue and address it accordingly.
- Incorrect Configuration: Make sure you configure your lint walker properly for your project. Using the wrong set of rules can lead to unnecessary warnings or missed issues.
By avoiding these common mistakes, you can get the most out of your lint walker and ensure that your code is as clean and efficient as possible.
The Future of Lint Walker
As technology continues to evolve, so too will lint walkers. We're already seeing advancements in AI-powered lint walkers that can provide even more detailed analysis of your code. These tools can not only catch errors but also suggest ways to optimize your code for better performance.
Additionally, as more and more companies move towards DevOps and continuous integration/continuous deployment (CI/CD) pipelines, lint walkers will become an integral part of the development process. They'll help ensure that code quality remains high throughout the entire development lifecycle.
So, whether you're just starting out as a developer or you're a seasoned pro, lint walkers are here to stay. Embrace them, use them wisely, and watch your code quality soar!
Kesimpulan
In conclusion, lint walkers are essential tools for any developer looking to write clean, efficient, and error-free code. They help prevent mistakes, improve code consistency, and make collaboration easier. By integrating lint walkers into your workflow and avoiding common mistakes, you can take your coding skills to the next level.
So, what are you waiting for? Start using a lint walker today and see the difference it can make in your development process. And don't forget to share this article with your fellow developers so they can benefit from the power of lint walkers too!

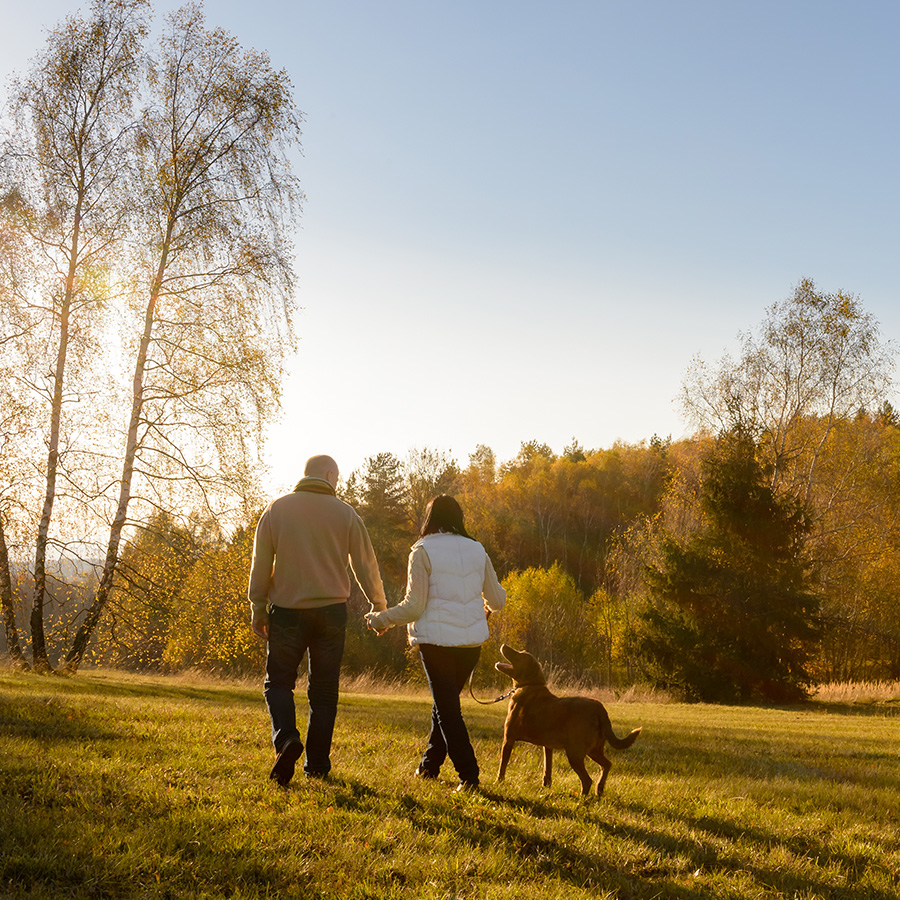
